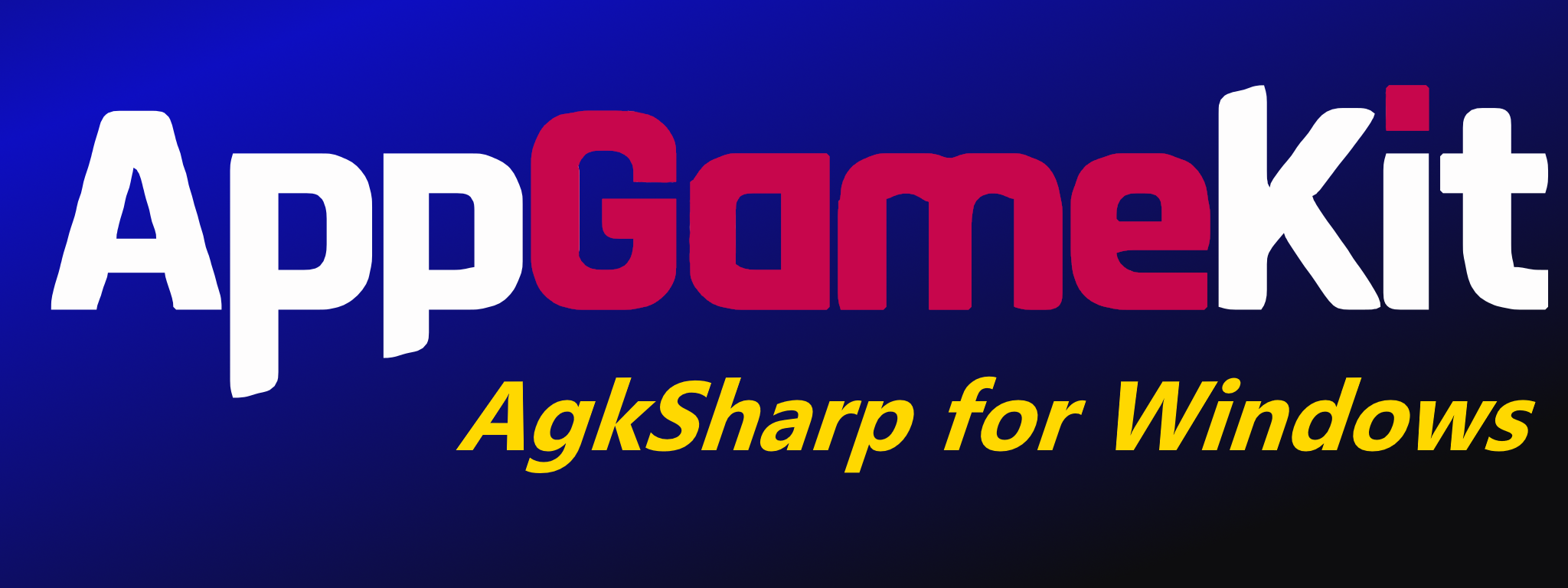
AgkSharp
A downloadable tool for Windows, macOS, and Linux
This project wraps the really easy to use game engine AppGameKit from TheGameCreators. So it can be used with C#. There is no scene editor or other editors, so it is a pure programmable engine. Is Unity, Unreal or Godot too complex and overwhelm you with functions. So AgkSharp is kept very simple and you can fully concentrate on programming.
The downloadable package contains template projects that you can copy and use. This library is available for Linux and Windows.
About AgkSharp for Windows
With AGKSharp you have the chance to write AppGameKit programs in the language C# and in VisualBasic. Now you can write your programs in an object-oriented language. I also provide a template that allows you to develop AppGameKit applications with windows-forms.
If you find AgkSharp helpful and you don't own AppGameKit yet, please buy AppGameKit. Let TheGameCreators know if you like AgkSharp. For now AgkSharp is only for Windows and Linux available.
What you Need?
- Download and install Visual Studio (AgkSharp was developed with Visual Studio 2017 but it work also with 2019).
- Download the Templates below.
Support you can be found in the AppGameKit forum from TheGameCreators. Here is also a good AppGameKit-Documentation.
If you like this project and would like to support it. I would be very happy about a donation.
Getting Started
- Download the template package below.
- To start a new project copy one of the templates into your development folder.
- Rename the template folder name and the '.csproj' file to your projectname.
- Open the '.csproj' file with Visual Studio.
- Right click the projectname in the VS envoriment and select the properties.
- Rename assamblyname and default namespace to your project-specific names.
- Now you can change the code to your wishes.
What does the code look like?
using System; using AgkSharp; using AGKCore; namespace AGKCore { static class AppInfo { public static string COMPANY_NAME = "My Company"; public static string WIN_TITLE = "AgkSharp_Template"; } } namespace AgkSharp_Template { static class Program { /// <summary> /// The main entry point for the application. /// </summary> [STAThread] static void Main() { Core.CreateWindow(AppInfo.WIN_TITLE, 640, 480, false); if (!Core.InitAGK()) return; Agk.SetVirtualResolution(320, 480); // display a background Agk.CreateSprite(Agk.LoadImage("media/background.jpg")); // create a sprite with ID that has no image Agk.CreateSprite(1, 0); Agk.SetSpritePosition(1, 130, 200); // add individual images into an animation list Agk.AddSpriteAnimationFrame(1, Agk.LoadImage("media/item0.png")); Agk.AddSpriteAnimationFrame(1, Agk.LoadImage("media/item1.png")); Agk.AddSpriteAnimationFrame(1, Agk.LoadImage("media/item2.png")); Agk.AddSpriteAnimationFrame(1, Agk.LoadImage("media/item3.png")); Agk.AddSpriteAnimationFrame(1, Agk.LoadImage("media/item4.png")); // play the sprite at 10 fps, looping, going from frame 1 to 5 Agk.PlaySprite(1, 10.0f, 1, 1, 5); // main loop while (Core.LoopAGK()) { Agk.Sync(); } Core.CleanUp(); } } }
The files that can be downloaded
AgkSharp_????????.zip
Contains the templates and binaries for Linux and Windows. The binaries are intended for when updates are released during project development. So only the DLL's have to be overwritten.
Examples.zip
Four examples translated from AppGameKit-Basic.
Dust2Dust.zip
An Asteroids clone. It is a game developed with AgkSharp and the corresponding project files (source code and media files) are attached. Most of the source code is documented.
Download
Click download now to get access to the following files:
Development log
- AgkSharp Update 2023-12-02Dec 02, 2023
- AgkSharp Update 2023-09-10Sep 10, 2023
- Update AgkSharp to version of AppGameKit 2022.09.28Nov 23, 2022
Comments
Log in with itch.io to leave a comment.
Is the project still being supported?
Very nice! So, what does the C# language offer over AGK's BASIC language? OOP constructs is probably the first thing that comes to my mind.
You may use either VB.NET or C# with AGKSharp. And either language provides you with all of the capabilities that the Visual Studio Development IDE has. Please remember that AGKSharp is an SDK for the AppGameKit Engine. As a result, you have greater flexibility than if you were to use AppGameKit itself. For example, you have access to the GUI for Windows Forms, though you have to do more work to integrate the event handling. You also can create DLLs using AGKSharp, allowing you to better modularize your project. I believe the only way you can do this with AppGameKit is with C++. You should note that AGKSharp does not come with much documentation but since it provides direct access to the AppGameKit Engine, the corresponding documentation for AppGameKit online should be more then helpful. As a result, you merely have to preface your AppGameKit API commands with "Agk.".
If you have any additional questions on this subject, please let me know...
Steve Naidamast (Sr. Software Engineer)
http://www.blackfalconsoftware.com
Very cool! I'm glad to see VB.Net is supported. 🤓

If you plan on using VB.NET with AgkSharp and would like to correspond with each other to discuss our projects, please let me know at my email... blackfalconsoftware@outlook.com
It is as snaidamast stated. In addition, you can use all the libraries that are available to C#.
If the network commands in AGK are not satisfactory then you have the possibility to use a library from C#.
Or using SQL databases, JSON and XML serialization. The possibilities are endless.